In this tutorial I will show you how to create a currency convertor using python program.
Install required Package:
pip install python-dateutil requests BeautifulSoup4
PYTHON CODE:
import requests from bs4 import BeautifulSoup as bs import re from dateutil.parser import parse def convert_currency_xe(src, dst, amount): url = f"https://www.xe.com/currencyconverter/convert/?Amount={amount}&From={src}&To={dst}" content = requests.get(url).content soup = bs(content, "html.parser") exchange_rate_html = soup.find_all("p")[2] last_updated_datetime = parse(re.search(r"Last updated (.+)", exchange_rate_html.parent.parent.find_all("div")[-2].text).group()[12:]) return last_updated_datetime, exchange_rate_html.text if __name__ == "__main__": from_currency = input("From Currency: ").upper() to_currency = input("To Currency: ").upper() amount = int(input("Enter the amount: ")) last_updated_datetime, exchange_rate = convert_currency_xe(from_currency, to_currency, amount) print("Last updated datetime:", last_updated_datetime) print(f"{amount} {from_currency} = {exchange_rate} {to_currency}")
OUTPUT:
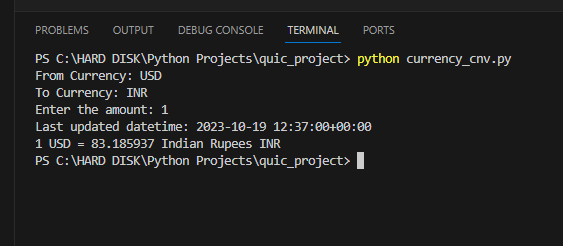
VIDEO GUIDE:
Post your comments / questions
Recent Article
- How to create custom 404 error page in Django?
- Requested setting INSTALLED_APPS, but settings are not configured. You must either define..
- ValueError:All arrays must be of the same length - Python
- Check hostname requires server hostname - SOLVED
- How to restrict access to the page Access only for logged user in Django
- Migration admin.0001_initial is applied before its dependency admin.0001_initial on database default
- Add or change a related_name argument to the definition for 'auth.User.groups' or 'DriverUser.groups'. -Django ERROR
- Addition of two numbers in django python
Related Article